Master Neural Networks for Predictive Analytics: Step-by-Step
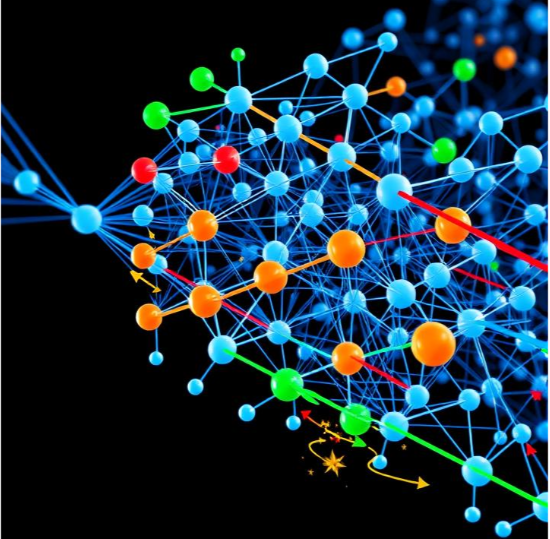
Learn how to build neural networks for predictive analytics with this detailed, step-by-step guide. Ideal for beginners and intermediates seeking to enhance their skills.
Neural Networks for Predictive Analytics
Neural networks have emerged as one of the most powerful tools for predictive analytics, leveraging complex algorithms to model and analyze data. In essence, predictive analytics involves examining historical data to forecast future outcomes, which can be particularly advantageous in fields such as finance, healthcare, marketing, and supply chain management.
The significance of using neural networks in predictive analytics lies in their capability to uncover intricate patterns within large datasets. Traditional statistical methods may struggle to capture non-linear relationships, whereas neural networks excel in this aspect. For instance, in finance, advanced models are applied to predict stock price movements based on numerous influencing factors, including historical trends, market sentiment, and economic indicators.
Throughout this comprehensive guide, a thorough understanding of how to build neural networks for predictive analytics will be laid out. Each step will be broken down in detail, ensuring clarity for even those who might be new to the concept. By the end of this guide, readers will not only grasp the theoretical underpinnings but also be equipped with practical skills to create their own predictive models.
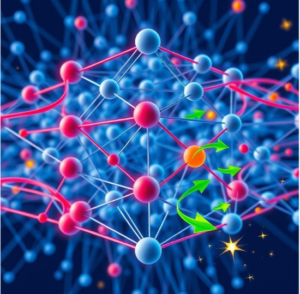
Understanding the Basics: Key Concepts of Neural Networks
Before diving into the intricacies of building neural networks, it is crucial to familiarize oneself with some fundamental concepts. Neural networks are algorithms designed to recognize patterns by mimicking the way human brains operate. They consist of interconnected layers of nodes, known as neurons, whereby each layer transforms the input data into progressively higher-level representations.
Neurons and Activation Functions
Each neuron processes data inputs and computes an output using an activation function. This function determines whether a neuron should be activated. Common activation functions include:
- Sigmoid , which squashes output to a range between 0 and 1, making it useful for binary classification tasks.
- Tanh provides outputs ranging from -1 to 1 and tends to perform better than the sigmoid function by centering outputs around zero.
- ReLU (Rectified Linear Unit) , the most widely used due to its efficiency in reducing computation time, outputs zero for negative inputs, while positive inputs pass through unchanged.
Architecture of Neural Networks
Neural networks are structured in layers, generally categorized as:
- Input Layer : This is where the data is fed into the neural network. Each neuron in this layer represents a feature from the dataset.
- Hidden Layers : Any layer between the input and output is classified as hidden. These layers transform inputs into outputs through various learned representations, with the number of hidden layers and the number of neurons per layer varying according to the complexity of the problem.
- Output Layer : This layer generates the final output. The number of neurons here corresponds to the number of classes for classification problems or a single neuron for regression tasks.
Understanding these components is essential for creating effective neural network models. As the network processes data, it learns the weight adjustments necessary to minimize the prediction error, a process called backpropagation.
Learning Rate and Loss Function
Two critical elements influencing model performance are the learning rate and loss function. The learning rate determines the size of steps taken during optimization — too high a rate may lead to overshooting the optima, while too low a rate can result in long training times. The loss function quantifies the difference between predicted and actual outcomes, guiding adjustments in weights.
Setting Up the Environment for Neural Network Development
A well-configured development environment is essential for successfully building and training neural networks. Python is the most popular programming language for data science and machine learning due to its simplicity and extensive library ecosystem.
Required Libraries
To start building neural networks, you need to install the following libraries:
- TensorFlow: A comprehensive open-source library developed by Google, offering flexible tools for building machine learning models.
- Keras: An API wrapped around TensorFlow that simplifies the process of building and training neural networks.
- NumPy: A powerful library for numerical computing, essential for efficiently handling arrays and matrices.
- Pandas: Ideal for data manipulation and analysis, especially for structured data.
- Matplotlib: Used for data visualization, aiding in understanding data patterns and model performance.
Installation Instructions
Follow these steps to set up your environment:
- Install Python: Download and install the latest version from python.org.
- Set up a Virtual Environment (Optional but Recommended):
- Install Libraries: Open your command-line interface and run:
- Set Up Jupyter Notebook: This interactive tool is beneficial for experimenting with code. Install Jupyter by running:
After completing these steps, you’ll have a functional environment for developing neural networks. You can launch Jupyter Notebook by typing jupyter notebook
in the command line, enabling the creation of .ipynb
files for a more user-friendly coding experience.
Data Collection and Preparation
The quality of data used in building neural networks greatly impacts model performance. While collecting relevant datasets requires effort, numerous repositories are available. Websites like Kaggle and the UCI Machine Learning Repository offer a wide range of datasets for various applications.
Data Acquisition
Identifying key features and target variables is fundamental. For example, if predicting house prices, features may include square footage, number of bedrooms, and location, while the target variable would be the house price.
Data Cleaning
Once the data is collected, cleaning it is crucial to ensure accuracy in model training. Common data cleaning tasks include:
- Handling Missing Values: Options include removing records, filling in averages or medians, or using advanced techniques like interpolation.
- Removing Duplicates: Duplicate records can skew data analysis, so unnecessary duplicates should be removed.
- Identifying Outliers: Outliers can negatively affect model training. Use statistical methods like the Z-score or IQR (Interquartile Range) to detect and manage them.
Data Normalization
After cleaning the data, normalization is the next step. Neural networks perform best when input values are scaled to a uniform range. Techniques include:
- Min-Max Scaling: Scales features to a range between 0 and 1.
- Standardization: Adjusts data based on z-scores, producing a mean of 0 and a standard deviation of 1.
Train-Test Split
It is standard practice to split datasets into training, validation, and test sets. The training set is used to train the model, the validation set for tuning hyperparameters, and the test set for final evaluation. A common distribution might be:
- 70% for training
- 15% for validation
- 15% for testing
Python’s Pandas library simplifies this task. Below is an example code snippet for data splitting:
By diligently following these steps, you can ensure that your data is well-prepared for building effective neural networks that yield accurate predictive analytics.
Building Your First Neural Network
With a clean and prepared dataset, you can begin building your first neural network. Using Keras, you can design complex architectures with minimal code. This section will guide you through constructing a basic feedforward neural network, also known as a multi-layer perceptron.
Creating the Model Architecture
Defining the model’s architecture is crucial when constructing a neural network. Keras’ sequential API allows you to build layers efficiently.
Here’s a step-by-step guide to building a simple neural network:
- Initialize the Model:
- Add Input Layer: The input layer size should match the number of features in your dataset.
- Add Hidden Layer(s): Add one or more hidden layers as necessary. More layers can capture complex patterns but may increase the risk of overfitting.
- Add Output Layer: The output layer should have neurons equal to the number of classes for classification problems or one neuron for regression.
Compiling the Model
After defining the architecture, the next step is compiling the model, specifying the optimizer, loss function, and performance metrics.
- Optimizer: ‘adam’ is widely used for its adaptability and efficiency.
- Loss function: Use ‘binary_crossentropy’ for binary classification or ‘categorical_crossentropy’ for multi-class classification.
With these components set, your model is ready for training.
Training the Neural Network
Once the model is prepared, the next step is training it using the training dataset. During this process, the model adjusts its weights and biases through forward propagation and backpropagation.
Training Process
To train the model, the .fit()
method is used, which requires several parameters, including the training data, target labels, epochs, and batch size.
- Epochs: This parameter indicates how many times the learning algorithm will pass through the entire training dataset. While more epochs can improve model performance, they can also increase the risk of overfitting.
- Batch Size: This defines the number of samples processed before the model’s internal parameters are updated. Smaller batch sizes may produce noisier gradients but can lead to better generalization.
Evaluating Neural Network Performance
Once the model training is complete, evaluating its performance on unseen data becomes crucial. The ultimate objective is to ensure the model not only performs well on training data but also generalizes to new, unseen data.
After training, evaluate the model using:
A confusion matrix provides deeper insights into classification performance:
Hyperparameter Tuning:
- Adjust the learning rate, apply dropout for regularization, and consider batch normalization to stabilize learning.
Real-World Applications
Neural networks find practical applications across industries:
- Healthcare: Predict patient outcomes and analyze imaging data.
- Finance: Assess credit risks, predict stock prices, and detect fraudulent transactions.
- Marketing: Enhance customer experience by predicting behavior and personalizing recommendations.
Optimizing Your Neural Network
The final stage in building a neural network is optimization, which involves fine-tuning various hyperparameters and potentially modifying the network’s architecture to enhance performance.
Hyperparameter Tuning
Key hyperparameters that significantly impact model performance include:
- Learning Rate: Adjusting the learning rate can greatly influence the speed of convergence and the overall performance of the model. Techniques like learning rate scheduling or Cyclical Learning Rates (CLR) can dynamically adjust the learning rate during training to improve results.
- Regularization Techniques: To prevent overfitting, methods like dropout, which randomly sets a portion of neurons to zero during training, are effective. You can use Keras’ Dropout layer to implement this:
- Batch Normalization: This technique helps stabilize the learning process by normalizing the inputs of each layer, allowing gradients to flow more effectively through the network. Batch Normalization can be added as follows:
Experimenting with Architecture
Testing different numbers of layers and neurons can also lead to performance improvements. The complexity of your model should match the complexity of the task at hand. Too few layers may fail to capture complex patterns, while too many layers can cause overfitting.
Lastly, implementing k-fold cross-validation provides a more comprehensive understanding of model performance by evaluating it across multiple splits of the training data. This technique helps ensure that the model generalizes well before finalizing it.
Implementing Neural Networks in Real-World Scenarios
Once a robust neural network has been developed, practical implementation becomes the focus. Neural networks equipped with predictive capabilities can be integrated into various industries, each yielding significant value.
Use Cases
- Healthcare : Neural networks are widely used to predict patient outcomes, from diagnosing diseases based on symptoms to analyzing imaging data. For instance, convolutional neural networks (CNNs) can detect anomalies in medical images, enhancing diagnostic capabilities.
- Finance : Many financial institutions deploy neural networks to assess credit risks, predict stock prices, or detect fraudulent transactions by analyzing transaction patterns.
- Marketing : Businesses adopt neural networks to enhance customer experience by predicting consumer behavior and personalizing product recommendations, ensuring that marketing strategies align effectively with customer preferences.
Deployment Options
When it comes to implementing trained neural networks in real-world scenarios, several deployment options are available:
- Cloud Services : Services like Amazon Web Services (AWS) and Google Cloud Platform offer infrastructure for deploying machine learning models globally. Utilizing platforms such as AWS SageMaker can streamline the deployment process.
- Real-time APIs : Converting trained models into APIs allows for real-time predictions. Using Flask or FastAPI in Python can facilitate this process, enabling developers to expose model predictions via web services.
Overall, effective implementation ensures greater organizational impact, optimizing resources while delivering results.
FAQs about Neural Networks and Predictive Analytics
Q1: What is a neural network?
A neural network is a computational model inspired by the human brain, consisting of interconnected nodes (neurons) organized in layers. These networks process data to identify patterns, enabling predictions based on input features.
Q2: How do neural networks learn?
Neural networks learn through backpropagation, adjusting weights based on calculated errors during the training phase. The learning process involves forward propagation, where inputs are transformed, and backward propagation, where errors are used to improve future predictions.
Q3: What types of problems can neural networks solve?
Neural networks can address various problems, including classification (e.g., spam detection), regression (e.g., housing price prediction), clustering (e.g., customer segmentation), and even content generation tasks, depending on architecture.
Q4: Do I need a lot of data to train a neural network?
While larger datasets generally produce better-performing models, smaller datasets can also yield effective models through techniques such as data augmentation, transfer learning, or leveraging synthetic data.
Q5: What is overfitting, and how can it be avoided?
Overfitting occurs when a model learns the training data too well, leading to poor performance on unseen data. Techniques like regularization, dropout, cross-validation, and monitoring validation performance can help mitigate overfitting.
Q6: How do I know if my neural network is working effectively?
Monitor performance metrics such as accuracy, precision, and recall during validation and testing phases. Visualizing results through confusion matrices or ROC curves can provide deeper insights into model efficacy.
Q7: Can neural networks be used for time-series forecasting?
Yes, recurrent neural networks (RNNs) and Long Short-Term Memory (LSTM) networks are specially designed to handle sequential data, making them ideal for time-series forecasting tasks.
Q8: What is the best way to start learning about neural networks?
Begin with foundational concepts in machine learning and neural networks. Use online platforms, courses, and hands-on practice with libraries like Keras or TensorFlow to develop practical skills.
Q9: Are neural networks the best approach for every predictive task?
While powerful, neural networks are not always the best solution. Simpler models such as linear regression or decision trees may be more suitable for less complex scenarios. The choice should be based on problem characteristics and dataset size.
Q10: What is the future of neural networks in predictive analytics?
As advancements in artificial intelligence continue, neural networks are expected to evolve further, enabling broader applications and improved outcomes across various disciplines. Trends include explainability, ethical AI, and integrating with IoT systems.
Building neural networks for predictive analytics involves a complex interplay of concepts and practices. This comprehensive guide has provided a roadmap from understanding fundamental concepts to practical implementation. Grasping these steps not only empowers individuals with the skills necessary for effective predictive modeling but also offers insights into the transformative impact of neural networks across various industries.
As the field of artificial intelligence continues to advance, continual learning and adaptation remain crucial. By investing in these efforts, one can cultivate expertise and contribute meaningfully to the rapidly evolving landscape of data science and predictive analytics.